WLL Rewards Core API
API Endpoint
api.rewards.wlloyalty.net/v1Introduction
The White Label Loyalty (WLL) Core Rewards API enables developers to create rich and fully customizable loyalty experiences for end-users through a simple to manage and update loyalty logic on the fly from WLL’s easy to use backend console.
Getting Started
Once you have received your API key you can begin using the API. The API key should be included in every request as the value of the X-Api-Key
header.
To report end-user interactions you must set up your system authentication configuration in the Loyalty Console. This can be any RS256 or HS256 JWT signing configuration (more info), WLL can supply the requisite OAuth infrastructure or your pre-existing authentication system can be used seamlessly.
Your API key applies some rate-limiting by default, specifically 100 requests per second with burst provision for 400 requests. We find this tends to be adequate for normal usage but speak to your account manager if you would like to discuss an adjustment.
API Host
Currently the WLL platform is available in two geographical regions, the EU and US. The API host to use is determined by the region of your tenant plus which environment you wish to connect to.
-
EU Production:
api.rewards.wlloyalty.net
-
EU Staging:
api.staging.rewards.wlloyalty.net
-
US Production:
api.rewards.us.wlloyalty.net
-
US Staging:
api.staging.rewards.us.wlloyalty.net
In all cases the APIs are identical, the only difference is that you should make your API calls to a different host.
API Testing
Click the button below to open an API testing workspace in Insomnia.
Once opened you should see a workspace with some pre-configured API requests available to test.
Some APIs require administrative authentication. You can should configure the workspace base environment options with your API key and admin auth credentials. View step 3 in this support document for more information.
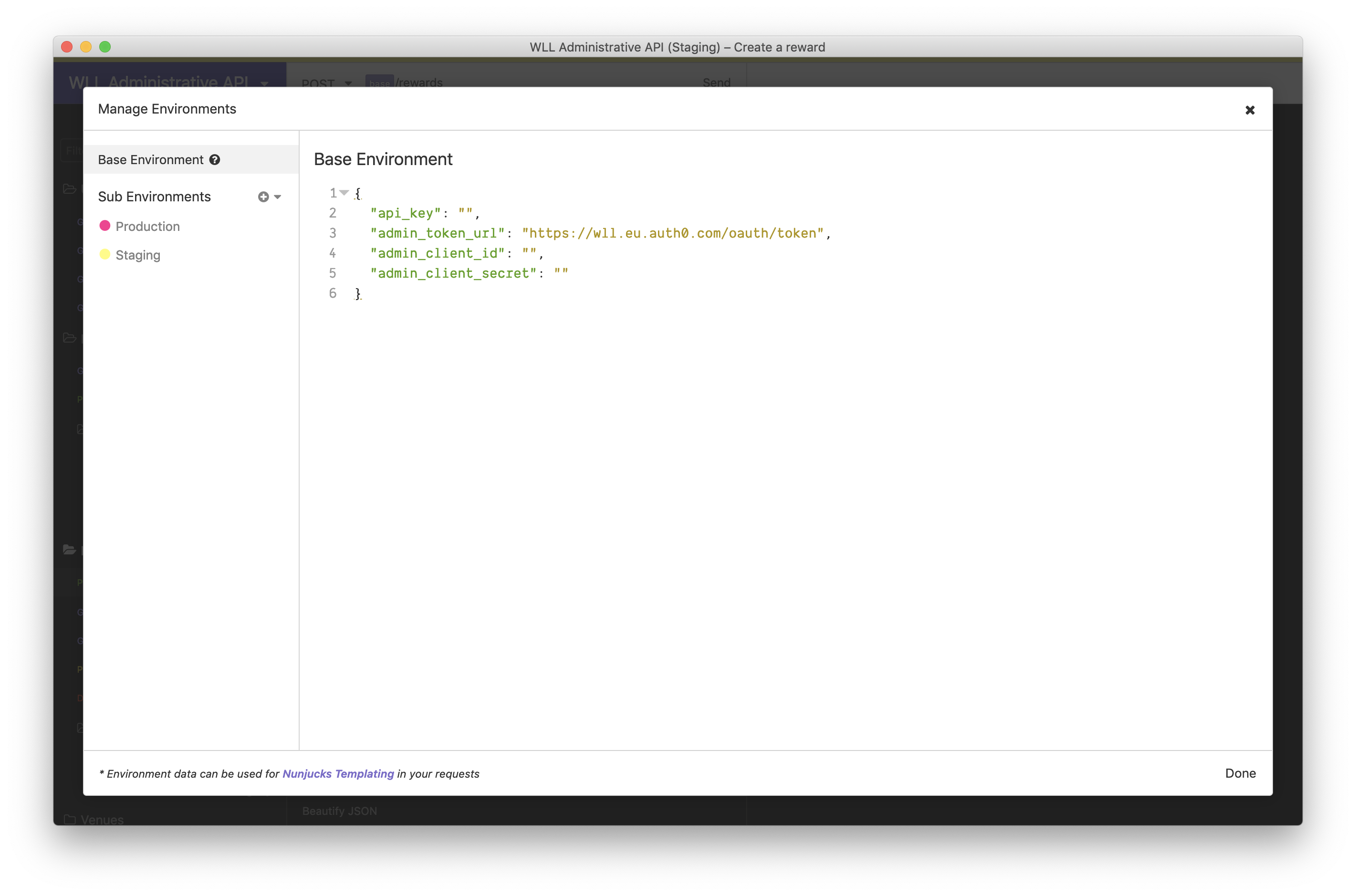
Authorization
All requests to the API must contain a X-Api-Key
header to identify your
tenant account. In addition to the API key, some methods additionally require
a Authorization
header to identify the user or entity accessing the API, and
ensure the requester has sufficient permissions for the requested operation.
This means that there are three levels of authorization which you should be aware when using this API:
-
🔑 Anonymous (API key only)
-
👤 User (API key + end-user auth token)
-
🔒 Admin (API key + administrative auth token)
Each API method described in this documentation will advise which type of authorization is requried.
Generating End-user Auth Tokens
The WLL API can be configured to recognize and validate the user auth tokens your customer-facing website or app already generates. This allows your customer-facing interfaces to query the WLL API directly as the specific logged-in user and only receive back data which the user is authorized to access.
WLL currently supports validating end-user auth tokens which conform to the following specification:
-
JWT structure per IETF RFC 7519
-
Algorithm value
RS256
(RSASSA-PKCS1-v1_5 with SHA-256) orHS256
(HMAC SHA-256) -
Mandatory payload claims:
sub
,iss
,iat
,exp
. -
If using
RS256
algorithm thekid
(Key ID) token header must be set to reference a public key accessible in your JSON Web Key Set (JWKS)
JWTs generated by any OIDC compliant authorization server is likely to meet this specification automatically. We have verified specifically that tokens generated by Auth0 and Firebase Auth work correctly.
If you wish to use this functionality please inform your success manager and provide the following details for configuration:
-
Algorithm:
HS256
orRS256
. -
Issuer: the value of the
iss
claim to expect. -
Shared Key: the symmetric key used to sign/validate the tokens if using HS256
-
JWKS URL: the URL where your JWKS can be accessed from if using RS256.
Integrating With Other Systems
The White Label Loyalty (WLL) platform models interactions between customer and business as a stream of events from which customer loyalty can be derived. The logic governing the loyalty programme can be defined and adjusted at any time via our cloud management console. Thus, the main work needed when integrating a system with WLL is to analyze what business events occur within the system and determine which events should be reported to WLL.
Codifying Events
The first step to integrating any system with the WLL platform is to codify the business events from which the loyalty programme logic will be derived. There are two parts to this step:
-
selecting which things happening during the day-to-day running of the business will be important to the loyalty programme,
-
planning the shape of the data payload which represents each of these things.
The codification of these events can be done via the WLL administrative console by creating Event Types. During the creation process you will be asked to provide a name to represent the event type throughout the platform plus a schema for validating any data payload sent as part of an event of this type. For clarity it is recommended that event type names should follow the principles outlined in the section below.
In addition to the taxonomical aspects of creating an event type you will also need to define whether it is a public or private event type. Private event types may only be reported by a secure server with access to a privileged secret. Whereas public event types are reported directly by authenticated users.
Kinds of Events
There are three main kinds of business events which you might want to codify and report to WLL.
Customer Actions
Customer actions are things which customers have themselves done during some interaction with the business. These kinds of events will almost always be public events since they are generally reported from the client side.
We recommend naming these event types in the form of a past-participle verb followed by a noun, such as VISITED_SHOP
, REDEEMED_CODE
, or ATE_SANDWICH
. In this way reporting an event can be thought in terms of a sentence “Jane Doe visited a shop” wherein the accompanying payload provide further detail.
Customer Proceedings
Customer proceedings are things which relate to a customer but haven’t been directly triggered by the customer. These events are usually triggered by a back-office business process. These kinds of events will almost always be private events since the source of truth for these things will usually be a secure backend server.
We recommend naming these event types in the form of a noun followed by a past-participle verb, such as INVOICE_RAISED
, REFUND_AWARDED
, CARD_INVALIDATED
. In this way reporting an event can be thought of in terms of a sentence “Jane Doe’s invoice was raised”.
Business Proceedings
Business proceedings are things which occur during the day-to-day running of the business but don’t relate to a specific customer.
Reporting Events
Once the business has decided on which events are relevant all events should be reported to the /events endpoint of the WLL Rewards API. Documentation on the format of this API call is here.
When reporting events you will likely want to supply a data payload for the WLL platform to capture. This data payload will enable you to customize the loyalty logic at a granular level. For example the number of points awarded to a user for an event could be configured to vary based on the time of day, the day of week, total spend, or any other data that is passed in the payload.
Examples
Transaction Completed
When a transaction is completed, the integrating system could report an event to the WLL platform similar to the below:
POST https://api.rewards.wlloyalty.net/events
Content-Type: "application/json"
X-Api-Key: $api_key
Authorization: Bearer $event_type_secret
{
"type": "TRANSACTION_COMPLETED",
"subject": "$user_id",
"payload": {
"transactionId": "12345",
"totalAmount": 100,
"purchaseChannel": "Online"
"itemsPurchased": [
{
"sku": "12345",
"quantity": 2
},
{
"sku": "67890",
"quantity": 1
}
]
}
}
Completed Survey
When a survey is completed, the integrating system could report an event to the WLL platform similar to the below:
POST https://api.rewards.wlloyalty.net/events
Content-Type: "application/json"
X-Api-Key: $api_key
Authorization: Bearer $event_type_secret
{
"type": "COMPLETED_SURVEY",
"subject": "$user_id",
"payload": {
"surveyId": "ABC123",
"questionsAnswered": 4,
"completionScore": 80
}
}
Events ¶
Test Reaction ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"type": "VISITED_VENUE",
"payload": {},
"subject": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"occurredAt": "2018-06-08T08:57:47.198Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type": {
"type": "string",
"description": "The name of the event-type being submitted."
},
"payload": {
"type": "object",
"properties": {},
"description": "The free-form data payload for this event."
},
"subject": {
"type": "string",
"description": "The authentication ID of the user who is the subject of this event."
},
"occurredAt": {
"type": "string"
}
},
"required": [
"type"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"totalPoints": 12,
"reactions": [
{
"type": "POINTS",
"points": {
"operation": "SUM",
"arguments": [
{
"type": "NUMBER",
"value": "12"
}
]
}
}
],
"activatedReactors": [
{
"name": "Example Reactor Name",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"totalPoints": {
"type": "number"
},
"reactions": {
"type": "array"
},
"activatedReactors": {
"type": "array"
}
},
"required": [
"totalPoints",
"reactions",
"activatedReactors"
]
}
},
"required": [
"status",
"data"
]
}
Test ReactionPOST/events/test_reaction
Use this endpoint to test what reactions would be triggered if some data was reported as an event.
The request body is identical to the request for reporting an event,
the primary difference is that this request must be made with normal admin authentication and the only way to test the reaction for a specific user is by including the subject
key explicitly.
The payload
must conform to the schema dictated by the event-type. If no schema is defined then the payload may be omitted.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:events
scope. Non-compliant requests will be rejected.
Events Collection ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"type": "VISITED_VENUE",
"payload": {}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type": {
"type": "string",
"description": "The name of the event-type being submitted."
},
"payload": {
"type": "object",
"properties": {},
"description": "The free-form data payload for this event."
}
},
"required": [
"type"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": 0,
"type": {
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
},
"subject": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"reportedAt": "2018-06-08T08:57:47.198Z",
"registeredAt": "2018-06-08T08:57:47.198Z",
"reactedAt": "2018-06-08T08:57:47.198Z",
"occurredAt": "2018-06-08T08:57:47.198Z",
"payload": {}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"type": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"isPrivate": {
"type": "boolean"
},
"schema": {
"type": "object",
"properties": {
"$schema": {
"type": "string"
},
"type": {
"type": "string"
},
"properties": {
"type": "object",
"properties": {}
},
"required": {}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"omitFromUserActivity": {
"type": "boolean"
},
"isActive": {
"type": "boolean"
}
},
"required": [
"id",
"name",
"description",
"isPrivate"
]
},
"subject": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"authIdentifier": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"isRestricted": {
"type": "boolean"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
}
},
"required": [
"id",
"accountNumber",
"authIdentifier",
"isRestricted",
"createdAt",
"updatedAt"
]
},
"reportedAt": {
"type": "string"
},
"registeredAt": {
"type": "string"
},
"reactedAt": {
"type": "string"
},
"occurredAt": {
"type": "string"
},
"payload": {
"type": "object",
"properties": {}
}
},
"required": [
"id",
"type",
"subject",
"reportedAt",
"payload"
]
}
},
"required": [
"status",
"data"
]
}
Report an EventPOST/events
Use this endpoint to pass back information about the interactions between end-users and the business. To understand more about what events are, see the “Codifying Events” section of the documentation.
This endpoint can be used in one of two ways; the first is that actions carried out directly by an authenticated user can be reported directly to the API for a public event-type.
The second mode of use is for interactions which occur indirectly to the end-user, in this case an event can be reported for a private event-type from a trusted environment using a secret token. This mode can be used to easily integrate 3rd-party systems.
The payload
must conform to the schema dictated by the event-type. If no schema is defined then the payload may be omitted.
You may optionally also include an additional timestamp property occurredAt
in your request to represent when this event actually occurred in the real world. This is useful in cases where you are reporting events after some delay.
Request bodies over 300kb will be rejected.
Authenticating the request
Private Event-types
To submit a private event the event-type’s own specific secret must be included as a bearer token in the Authorization
header.
This secret is generated when the event-type is created and can be retrieved via the WLL Admin Console. It is also possible to retrieve programmatically if required.
When reporting events in this manner you must pass the subject
field which corresponds to the loyalty actor who is the subject of this event. The subject
value may be any one of:
-
user.authIdentifier
-
user.id
-
user.accountNumber
-
team.id
-
team.accountNumber
externalIdentifier
is not supported for this since it is not guaranteed to be unique.
Public Event-types
To submit a public event the authenticated user’s personal access token should be included in the Authorization
header instead.
It is not possible to pass a custom subject
for a public event-type, since the subject will be automatically assigned based on the authenticated user.
Duplicate Detection
This endpoint will return a 409 “Event already exists” error if an identical event has already been reported in the past 60 seconds. An event is considered identical if it has the same type, subject, and payload.
🔐 Multiple authorization modes
Requests to this method require one of two authorization types depending on the kind of event being submitted. Details are explained above. Non-compliant requests will be rejected.
N.B. Authorization for private events is a special case not used elsewhere.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Content-Range: event 0-1/2
Body
{
"status": "success",
"data": [
{
"id": 0,
"type": {
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
},
"subject": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"reportedAt": "2018-06-08T08:57:47.198Z",
"registeredAt": "2018-06-08T08:57:47.198Z",
"reactedAt": "2018-06-08T08:57:47.198Z",
"occurredAt": "2018-06-08T08:57:47.198Z",
"payload": {}
},
{
"id": 0,
"type": {
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
},
"subject": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"reportedAt": "2018-06-08T08:57:47.198Z",
"registeredAt": "2018-06-08T08:57:47.198Z",
"reactedAt": "2018-06-08T08:57:47.198Z",
"occurredAt": "2018-06-08T08:57:47.198Z",
"payload": {}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all EventsGET/events
Get a list of all the events which have been reported for your tenant.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:events
scope. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- Parameters + limit: 10 (number, optional)
The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000. + skip: 0 (number, optional)
The number of items to skip before selecting. + sort: id (enum[string], optional)
The property to use for sorting. + Members + id + occurredAt + reportedAt + registeredAt + reactedAt + order: ASC (enum[string], optional)
The order to sort items. + Members + ASC + DESC + filter:
reportedAt:(gt:'2019-01-01T00:00:00Z')
(optional) O-Rison encoded filter string
Individual Event ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": 0,
"type": {
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
},
"subject": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"reportedAt": "2018-06-08T08:57:47.198Z",
"registeredAt": "2018-06-08T08:57:47.198Z",
"reactedAt": "2018-06-08T08:57:47.198Z",
"occurredAt": "2018-06-08T08:57:47.198Z",
"payload": {}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"type": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"isPrivate": {
"type": "boolean"
},
"schema": {
"type": "object",
"properties": {
"$schema": {
"type": "string"
},
"type": {
"type": "string"
},
"properties": {
"type": "object",
"properties": {}
},
"required": {}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"omitFromUserActivity": {
"type": "boolean"
},
"isActive": {
"type": "boolean"
}
},
"required": [
"id",
"name",
"description",
"isPrivate"
]
},
"subject": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"authIdentifier": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"isRestricted": {
"type": "boolean"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
}
},
"required": [
"id",
"accountNumber",
"authIdentifier",
"isRestricted",
"createdAt",
"updatedAt"
]
},
"reportedAt": {
"type": "string"
},
"registeredAt": {
"type": "string"
},
"reactedAt": {
"type": "string"
},
"occurredAt": {
"type": "string"
},
"payload": {
"type": "object",
"properties": {}
}
},
"required": [
"id",
"type",
"subject",
"reportedAt",
"payload"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve an EventGET/events/{id}
Retrieve the details of a single Event.
There are four timestamps recorded on each event, the purpose of each is described below:
-
occurredAt
: when did this event actually take place in reality? This will only contain a value if it was provided when the event was reported. -
reportedAt
: when was this event initially reported to WLL. -
registeredAt
: when was this event stored in WLL’s database, in effect this means when were points (if any) granted for this event. -
reactedAt
: when were asynchronous reactions such as webhooks processed for this event.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:events
scope. Non-compliant requests will be rejected.
- id
number
(required) Example: 16389ID of the Event.
Event Reactions ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"totalPoints": 12,
"reactions": [
{
"type": "POINTS",
"points": {
"operation": "SUM",
"arguments": [
{
"type": "NUMBER",
"value": "12"
}
]
},
"reactorName": "Example Reactor Name"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"totalPoints": {
"type": "number"
},
"reactions": {
"type": "array"
}
},
"required": [
"totalPoints",
"reactions"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve Reaction InformationGET/events/{id}/reactions
Retrieve information about the reactions which occurred for a given event.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
- id
number
(required) Example: 16389ID of the Event.
Event Types ¶
Event-Type Collection ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List All Event TypesGET/event_types
Get a list of all the event-types which have been configured for your tenant. Private event-types will not report their secret, this must be retrieved securely via the Loyalty console.
Properties
-
omitFromUserActivity :
false
(boolean):When authorized as an admin this field is ignored, when authorized as a user however this field decides whether an event with this event-type is shown in the user activity.
Individual Event-Type ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "d7efcfb2-dfe3-47a7-b826-4ef8b9b0012f",
"name": "COMPLETED_EXAMPLE_ACTION",
"description": "",
"isPrivate": false,
"schema": {
"$schema": "http://json-schema.org/draft-06/schema#",
"type": "object",
"properties": {},
"required": []
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"omitFromUserActivity": false
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"isPrivate": {
"type": "boolean"
},
"schema": {
"type": "object",
"properties": {
"$schema": {
"type": "string"
},
"type": {
"type": "string"
},
"properties": {
"type": "object",
"properties": {}
},
"required": {}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"omitFromUserActivity": {
"type": "boolean"
},
"isActive": {
"type": "boolean"
}
},
"required": [
"id",
"name",
"description",
"isPrivate"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve an Event TypeGET/event_types/{identifier}
Retrieve the details of a single EventType.
You specify either the UUID of the event-type or the name of the event-type in the URL.
Properties
-
omitFromUserActivity :
false
(boolean):When authorized as an admin this field is ignored, when authorized as a user however this field decides whether an event with this event-type is shown in the user activity.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with read:event_types
scope. Non-compliant requests will be rejected.
- identifier
string
(required) Example: EXAMPLE_NAMEname or ID of the event-type to retrieve.
Users ¶
Users Collection ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "your custom identifier",
"authIdentifier": "unique external user ID",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
}
},
"required": [
"isRestricted",
"profile"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Register a New UserPOST/users{?locale}
Register a new user on the system with the profile data passed in the request body.
This endpoint can be used in one of two ways: as an administrative action or as a user action.
The mode is dependent on the token passed in the Authorization
header.
If the token is found to be administrative the authIdentifier
property must be passed so that the user can in future be identified from their authentication token.
Otherwise the token will be checked against the tenant authentication configuration. In this case the authIdentifier
will be derived from the sub
claim of the passed JWT.
Fields
externalIdentifier
can be used to attach any arbitrary string to the user, this is sometimes useful when linking this representation of the user to one in a separate system. This field is not checked for uniqueness but it cannot be more than 512 characters in length.
authIdentifier
is required when creating a user in administrative mode to associate the account with the ID used by the tenant authentication server.
If the API will never be directly called with a user authentication token, this field can be an arbitrary unique string and may be used instead of externalIdentifier
. This field will be ignored if the endpoint is invoked in user mode.
isRestricted
is intended to be used for GDPR workflows where the end-user requests restriction of further processing of their data. This must be false
when a new account is being created since if the user does not give permission for their data to be processed an account cannot be created.
linkedVenues
can be used to provide a list of venues which this user should be associated with. The array should contain an object with the venue ID as a property.
profile.familyName
the user’s family name (surname, last name):
Required; string; between 1 and 64 characters in length.
profile.givenName
the user’s given name (first name):
Required; string; between 1 and 64 characters in length.
profile.emailAddress
the user’s email address:
Required; string; email format.
profile.gender
the user’s gender, if not provided it will default to UNKNOWN
:
Optional; enum (UNKNOWN, MALE, FEMALE, OTHER).
profile.country
the user’s country of residence:
Optional; string; ISO 3166-1 alpha-2 format.
profile.city
the user’s city of residence:
Optional; string; between 1 and 256 characters in length.
profile.postcode
the user’s postcode:
Optional; string; between 1 and 128 characters in length.
profile.pictureUrl
the HTTPS URL for a picture to represent the user:
Optional; string; HTTPS URLs only.
profile.attributes
optional arbitrary map of keys and values:
Optional; object; Any JSON key-values.
Handling Consent
consentsDefinition
array of consents given by user: Optional; array of consent objects
Consent objects take the following form:
{
"type": { "id": "9cb2cc08-e033-4200-86a7-95a03ba09462" },
"checked": true
}
The type.id
refers to the specific pre-configured consent type which the user has given.
checked
is a boolean value to specify whether the user has given this type of consent.
Handling Referrals
referralInviteCode
optional the invite code or link this user used during registration. See the Referral Campaign section for more info on this flow.
If provided, the referralActivity
property must be provided as well.
referralActivity
optional contains data that explains the new user’s referral journey, which can later help with attribution.
If provided, the referralInviteCode
property must be provided as well.
Of note are three properties, source
, sourceUserId
and activityType
.
source
and sourceUserId
describe the touch point through which the referral link was originally shared and clicked on. Eg: the referral code was shared via email to the email address ‘abc123@xyz.com’, making EMAIL as the source
and ‘abc123@xyz.com’ as the sourceUserId
. If this data isn’t available, simply passing a device id of the new user as sourceUserId
, and OTHER as source
is OK.
activityType
describes whether this attribution data is about a CLICK or a REGISTER activity. For the purposes of a new user’s registration, activityType
should be REGISTER.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization
Details are explained above. Non-compliant requests will be rejected.
- locale
string
(optional) Example: enThe active locale of the user - used to capture the releavant consent description.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all UsersGET/users{?limit,skip,sort,order,filter}
Get a list of all the users registered in your tenant.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: idThe property to use for sorting.
Choices:
id
createdAt
updatedAt
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: isRestricted:(eq:!f)O-Rison encoded filter string
Specific User ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve Specific UserGET/users/{id}
Retrieve the details of the user specified by their ID.
The ID parameter can be any one of: id
, authIdentifier
, or accountNumber
.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User to retrieve.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Update Specific UserPATCH/users/{id}
Update the details of the user specified by their ID.
The ID parameter can be any one of: id
, authIdentifier
, or accountNumber
.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User to update.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"affected": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"affected": {
"type": "number"
}
}
}
},
"required": [
"status",
"data"
]
}
Delete Specific UserDELETE/users/{id}
Delete a specific user by their ID. Note that this operation deletes the profile
data only. The top-level identifier information is retained to prevent conflicts
The ID parameter can be any one of: id
, authIdentifier
, or accountNumber
.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User to retrieve.
Active User ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve Active UserGET/users/me
Retrieve the details of the currently authenticated user.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Update Active UserPATCH/users/me
Update the details of the currently authenticated user.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Specific User wallet ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"points": 0,
"tier": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold"
},
"lifetimeEarn": 0,
"loyaltyCost": 0,
"vouchers": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"tier": {
"type": [
"object",
"null"
],
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
}
}
},
"lifetimeEarn": {
"type": "number"
},
"loyaltyCost": {
"type": "number"
},
"vouchers": {
"type": "array"
}
},
"required": [
"points",
"lifetimeEarn",
"loyaltyCost"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve Specific WalletGET/users/{id}/wallet
Retrieve the loyalty wallet of the user specified by their ID.
The ID parameter can be any one of: id
, authIdentifier
, or accountNumber
.
Vouchers returned in the response have two timestamps of interest:
createdAt
: this timestamp represents when the user purchased or otherwise received the voucher.redeemedAt
: this timestamp represents when the user initiated the redemption flow for the voucher.
The redeemedAt
property will be null if the voucher has not yet been redeemed.
While the voucher is unredeemed the code
property will also be null.
Once the voucher is redeemed by the user, subsequent requests to this endpoint will reveal the code object.
Once the redemption has expired or has been explicitly validated the voucher will disappear from this endpoint’s response.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with at least read:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User for whom the wallet should be retrieved.
Active User Wallet ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"points": 0,
"tier": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold"
},
"vouchers": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"tier": {
"type": [
"object",
"null"
],
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
}
}
},
"vouchers": {
"type": "array"
}
},
"required": [
"points"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve Active WalletGET/users/me/wallet
Retrieve the loyalty wallet of the currently authenticated user.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Active User Purchases ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"resource": {
"type": "REWARD",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"resource": {
"type": "REWARD",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
},
"benefit": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
},
"completedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"resource": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"REWARD",
"WEGIFT_PRODUCT"
]
},
"id": {
"type": "string"
}
},
"required": [
"type",
"id"
]
},
"benefit": {
"type": "object",
"properties": {
"type": {
"type": "string"
},
"id": {
"type": "string"
}
},
"required": [
"id"
]
},
"completedAt": {
"type": "string"
}
},
"required": [
"resource",
"completedAt"
]
}
},
"required": [
"status",
"data"
]
}
Active User PurchasePOST/users/me/purchases
❌ This API is Deprecated
This API is deprecated and will be removed in the future. Use /commands/purchase_reward/
instead.
Use this endpoint to trigger a purchase of the provided resource for the currently authenticated user.
In the request body you should specify the ID of the reward which the user wishes to purchase. The response will vary according to the type of resource granted by the purchase.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Specific User Purchases ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"resource": {
"type": "REWARD",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"resource": {
"type": "REWARD",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
},
"benefit": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
},
"completedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"resource": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"REWARD",
"WEGIFT_PRODUCT"
]
},
"id": {
"type": "string"
}
},
"required": [
"type",
"id"
]
},
"benefit": {
"type": "object",
"properties": {
"type": {
"type": "string"
},
"id": {
"type": "string"
}
},
"required": [
"id"
]
},
"completedAt": {
"type": "string"
}
},
"required": [
"resource",
"completedAt"
]
}
},
"required": [
"status",
"data"
]
}
Specific User PurchasePOST/users/{id}/purchases
❌ This API is Deprecated
This API is deprecated and will be removed in the future. Use /commands/purchase_reward/
instead.
Use this endpoint to trigger a purchase of the provided resource for the specific user with the provided ID.
In the request body you should specify the ID of the reward which the user wishes to purchase. The response will vary according to the type of resource granted by the purchase.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User for whom the wallet should be retrieved.
Active User Redemptions ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"resource": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
},
"position": {
"longitude": 170.9021,
"latitude": 80.9021
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"resource": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"resource": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER"
]
},
"id": {
"type": "string"
}
},
"required": [
"type",
"id"
]
}
},
"required": [
"resource"
]
}
},
"required": [
"status",
"data"
]
}
Trigger RedemptionPOST/users/me/redemptions
Use this endpoint to trigger a redemption of the provided resource for the currently authenticated user.
The position
property is optional, if it is provided it will be used to calculate any defined Venues within a 1km radius of the position and include that information in the resulting redemption event payload.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Bulk User Summary ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "2096817f-2d8f-4690-bbcc-71868ad1f3c9",
"accountNumber": 123001,
"authIdentifier": "d40fd452-ec8c-45c3-afa8-b2d23f9ebd87",
"externalIdentifier": "33df89cd-851e-4ef3-be5f-c0f1920b181b",
"wallet": {
"userLifetimePoints": 3100,
"currentYearPoints": 1900,
"lastYearPoints": 1200,
"currentMonthPoints": 900,
"lastMonthPoints": 1900,
"last24HoursPoints": 0,
"points": 1900,
"correctAsOf": "2024-11-16T05:57:33.500Z"
},
"pointsExpiryPrediction": {
"overallSummary": {
"type": "EXPIRY",
"summary": [
{
"pointsDueToExpire": 0,
"timePeriod": 1
},
{
"pointsDueToExpire": 0,
"timePeriod": 7
},
{
"pointsDueToExpire": 0,
"timePeriod": 30
},
{
"pointsDueToExpire": 0,
"timePeriod": 90
},
{
"pointsDueToExpire": 0,
"timePeriod": 365
}
]
}
},
"pointsSpendPrediction": {
"overallSummary": {
"type": "MAX_POINTS",
"summary": {
"pointsCap": 1000,
"remainingPoints": 900,
"percentageUsed": 10
}
}
},
"pointsEarnPrediction": {
"overallSummary": {
"type": "EXPIRY",
"summary": [
{
"pointsDueToExpire": 0,
"timePeriod": 7
}
]
}
},
"audiences": [
{
"id": "ec9706be-6000-4968-9ce5-bedc73f05a12",
"name": "Users with over 200 points."
}
],
"tier": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": ""
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
Bulk User SummaryGET/users/summary
Use this endpoint to fetch a list of users summaries.
Account details include: id
, accountNumber
, authIdentifier
and externalIdentifier
.
The wallet
contains information about the user’s points.
pointsExpiryPrediction
shows how many days are left before the user’s points expire.
pointsSpendPrediction
and pointsEarnPrediction
indicate how many points the user has spent and earned, respectively.
These prediction metrics are updated daily just after 00:00 UTC, and are available for the following time periods, in days: (day, week, month, quarter, and year).
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
Audiences ¶
Audience Results ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List Audience ResultsGET/audiences/{id}/results
Retrieve a list of users who met the criteria of this audience when last calculated.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with read:audiences
and read:users
scopes
Non-compliant requests will be rejected.
If a user token is provided rather than an admin token (or if the impersonate header is sent with an admin token), this endpoint will only return results which this user should have visibility of – namely their own result. This behaviour can be used from the client-side to check if the current user is a member of a specific audience.
Note: this request does not invoke a synchronous calculation of audience membership, it returns the results of async audience calculations. The timing of the last calculation depends on the configured frequency of this audience. This endpoint should be used where eventual consistency is appropriate.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the audience.
Rewards ¶
Understanding Rewards and Benefits
In the terminology of the WLL platform a “Reward” is a description of some real-world value which a end-user could get. It has two main functions:
- providing a container for marketing assets such as images, descriptions, terms and conditions.
- specifying the requirements for an end-user to get it, e.g. points cost, tier status, audience membership.
There are multiple types of reward which reflect the various methods for delivering value to end-users. All rewards (except static offers) support the purchase method which ensures the user meets the requirements for the reward and deducts any points necessary from the user’s balance.
Some types of reward generate a “Benefit” as a result of purchasing the reward. Benefits are uniquely linked to the purchasing user, they provide a means for the user to actually get some real-world value. An analogy which may be helpful for readers familiar with software: a Reward is similar to a “class”, and a Benefit is an instance of that class.
There is a different type of benefit corresponding to each different type of reward which generates benefits. Broadly the types of benefit can be categorized into Vouchers and Gift Cards.
Voucher benefits are fulfilled by a system external to WLL, typically a ePOS, eCommerce, or shop staff member. They function by assigning a voucher code with an expiry date to that user during the purchase which can only be accessed by calling the “redemption” method.
With Gift Card benefits, WLL handles fulfilment on your behalf, there are three options for fulfilment: direct email, URL, or raw text code. Some brands only support one or the other of those options, but there’s plenty of choice so you can choose the delivery method that works best for you and then just select the brands which support that method.
Rewards Collection ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"price": 0,
"priority": 0,
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"tier": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
},
"discounts": [
{
"type": "FIXED",
"value": 1000,
"sku": "321ZA",
"productSku": "AZ123"
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all RewardsGET/rewards{?limit,skip,sort,order,filter}
Get a list of all rewards which your token is authorized to view.
By default this endpoint will return all rewards which are available at the time of the request.
Importantly that means it will not exclude the rewards which have been marked as “unpurchasable”.
To exclude such rewards you can supply the following query ?filter=purchasable:(eq:!t)
.
The exact list of rewards returned also depends on who is making the request, since rewards can be configured to only be visible to certain users.
Rewards may also have a isLocked
boolean property which indicates whether the reward is locked for purchasing for the active user.
This behaviour is controlled by the “purchasable by audiences” configuration of the reward, where if the requesting user is not a member of one of the configured allowable audiences the isLocked
property will be set to true
.
Note: if the reward is not locked for the requesting user, the isLocked
property will be omitted rather than explicitly set false
.
Visibility skip mode
As mentioned above, if the request is from an end-user’s perspective, some of the rewards may be configured to be invisible for that user.
By default, invisible rewards will still count towards the requested limit
even though they won’t be included in the response body.
This behaviour may not be desirable if you are expecting a consistent number of items in the response body.
To support that use case there is an optional query parameter you can pass in the request to change the behaviour: completelySkipInvisible
.
If you pass this parameter only visible rewards (i.e. ones returned in the response body) will count towards the limit
.
This behaviour is an opt in because it is less performant than the default and so may result in increased latency.
Content-Range and pagination
As with all WLL API list endpoints the response Content-Range header indicates what range of the whole set of items were returned in the response body.
Given ?skip=0&limit=10
request parameters the response range will look like:
reward 0-9/100
Where
-
0
is the index of the first item considered for inclusion in the response body, -
9
is the index of the last item considered for inclusion in the response body, -
100
is the total number of items in the entire set.
These indices do not always indicate the total number of items returned in the body, merely what range of items was considered for inclusion.
Whereas, given ?skip=0&limit=10&completelySkipInvisible
the response range may look like:
reward 0-13/*
The obvious difference is that in this case the total number of items in the entire set is not given to indicate that you cannot know definitively how many further pages of items are available since it’s not known how many items will be visible to the user.
The other difference demonstrated here is the range is not determined by the limit
value because we have asked
to automatically skip any invisible items so as to fill the requested limit.
In this example, 4 of the first 10 rewards considered for inclusion were invisible to the user, so to fill the requested limit
the API had to move on to items 10, 11, 12, and 13.
Summary: Content-Range specifies what range of the overall set was considered for inclusion in the response – it does not specify the number of items returned.
In either case, to request the next page of rewards you should specify skip
based on the last index given in the previous response’s Content-Range, plus one.
For example, to request the second page of the first example above you would request ?skip=10&limit=10
.
To request the second page of the second example above you would request ?skip=14&limit=10
.
Requesting rewards as an end-user
There are two ways to retrieve the list of rewards which are available to a specific user:
Passing the User’s auth token
You can simply pass the user’s auth token in the Authorization
header of the request.
This approach is ideal when the request is coming directly from a user’s device and they have an active auth session.
See this section of documentation for more information about user auth tokens.
User impersonation with an admin token
You can alternatively pass the X-Impersonate
header in the request with the relevant user’s WLL ID.
You can only use this impersonation header when authorizing the request with an admin token which has read:users
permission.
Non-venue rewards
To return only the rewards which aren’t assigned to a particular Venue, filter for a null value on the venues
field e.g. venues:(eq:!n)
.
🔐 Multiple authorization modes
Requests to this method can use any of the following:
🔑 Anonymous (API key only)
👤 User Authorization
🔒 Admin Authorization
If using admin authorization the token must have at least read:rewards
permission; read:users
permission is also required if applying user impersonation.
The behaviour of this method will vary based on the authorization method, please see main description above for more information.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- completelySkipInvisible
string
(optional)Opt in to special mode described here.
Parameter values below are allowed but not required, merely including the param name will enable the mode.
Choices:
1
0
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: priorityThe property to use for sorting.
Choices:
priority
price
value
name
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: price:(gt:10)O-Rison encoded filter string
Individual Reward ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"price": 0,
"priority": 0,
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"tier": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
},
"discounts": [
{
"type": "FIXED",
"value": 1000,
"sku": "321ZA",
"productSku": "AZ123"
}
],
"description": "Redeem this reward for a free cup of coffee",
"terms": "",
"purchasableForAudiences": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"venues": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER",
"GENERIC_OFFER",
"AWIN_PROGRAMME",
"FIDEL_OFFER",
"OLIVE_OFFER",
"WEGIFT_PRODUCT",
"XOXO_PRODUCT"
]
},
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"purchasable": {
"type": "boolean"
},
"summary": {
"type": "string"
},
"defaultLocale": {
"type": "string",
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"price": {
"type": "number"
},
"priority": {
"type": "number"
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"tier": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
}
},
"required": [
"id",
"name",
"priority"
]
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
}
},
"discounts": {
"type": "array"
},
"description": {
"type": "string"
},
"terms": {
"type": "string"
},
"purchasableForAudiences": {
"type": "array"
},
"venues": {
"type": "array"
}
},
"required": [
"type",
"id",
"name",
"pictureUrl",
"purchasable",
"defaultLocale",
"createdAt",
"updatedAt",
"price",
"priority",
"availability",
"discounts",
"description",
"terms"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a RewardGET/rewards/{id}
Retrieve the details of a single Reward.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the reward.
Rewards Categories ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all Reward CategoriesGET/reward_categories{?limit,skip,sort,order,filter}
Get a list of all defined reward categories.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: priorityThe property to use for sorting.
Choices:
id
createdAt
updatedAt
name
priority
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: priority:(gt:10)O-Rison encoded filter string
Headers
X-Api-Key: $api_key
Body
{
"name": "Winter Offers",
"description": "Check out our exciting winter offers!",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"defaultLocale": "en",
"details": [
{
"locale": "en",
"name": "High Earners",
"description": "This is a description",
"pictureUrl": "https://google.com/logo.gif"
}
]
}
Schema
{
"type": "object",
"properties": {
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"defaultLocale": {
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
},
"details": {
"type": "array",
"items": {
"type": "object",
"properties": {
"locale": {
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
},
"required": [
"locale",
"name"
]
}
}
},
"required": [
"defaultLocale",
"details"
],
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
}
}
},
"required": [
"status",
"data"
]
}
Creating Reward CategoriesPOST/reward_categories
API to create a Reward Category
Specific Reward Category ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"name": "Winter Offers",
"description": "Check out our exciting winter offers!",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"defaultLocale": "en",
"details": [
{
"locale": "en",
"name": "High Earners",
"description": "This is a description",
"pictureUrl": "https://google.com/logo.gif"
}
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
}
}
},
"required": [
"status",
"data"
]
}
Update a Reward CategoryPATCH/reward_categories/{id}
Update the details of the reward category specified by their ID.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with update:rewards
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Reward Category to update.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
}
}
},
"required": [
"status",
"data"
]
}
Delete a Reward CategoryDELETE/reward_categories/{id}
The reward category will be deleted. Once deleted the reward category is not accessible. (It is not archived)
Note. Before the category can be deleted all associated rewards must be unlinked first.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with update:rewards
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Reward Category to delete.
Benefits ¶
Benefits Collection ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
},
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": "2018-06-10T21:07:01.219Z",
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
},
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "XOXO_PRODUCT",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"orderId": "123456",
"pin": "123ABC",
"url": "https://docs.whitelabel-loyalty.com/assets/gift-card-redemption.html",
"activationKey": "JS9Y-4TVB",
"isDelayed": false
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all BenefitsGET/benefits{?limit,skip,sort,order,filter}
Get a list of all benefits within your tenant. Benefits are the output of a reward purchase. There are different types of benefits each corresponding to a different type of reward as explained here.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization
Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: createdAtThe property to use for sorting.
Choices:
createdAt
redeemedAt
expiresAt
reward.*
owner.*
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Individual Benefit ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"type": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"redeemedAt": {
"type": [
"string",
"null"
]
},
"expiresAt": {
"type": [
"string",
"null"
]
},
"reward": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER",
"GENERIC_OFFER",
"AWIN_PROGRAMME",
"FIDEL_OFFER",
"OLIVE_OFFER",
"WEGIFT_PRODUCT",
"XOXO_PRODUCT"
]
},
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"purchasable": {
"type": "boolean"
},
"summary": {
"type": "string"
},
"defaultLocale": {
"type": "string",
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
}
},
"required": [
"type",
"id",
"name",
"pictureUrl",
"purchasable",
"defaultLocale"
]
},
"owner": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"authIdentifier": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"isRestricted": {
"type": "boolean"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
}
},
"required": [
"id",
"accountNumber",
"authIdentifier",
"isRestricted",
"createdAt",
"updatedAt"
]
},
"burnedAt": {
"type": [
"string",
"null"
]
}
},
"required": [
"id",
"type",
"reward"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a BenefitGET/benefits/{id}
Retrieve the details of a specific Benefit by its ID.
The core data structure for all Benefits is the same, but each type of benefit extends the core data structure with its own specific properties. Additionally for some types of Benefit, confidential information will not be returned in the response until it has been redeemed. For example, a Voucher benefit will not give it’s code until it has been redeemed.
Types of Benefit
Voucher
Vouchers provide a mechanism for you to issue codes to users which can be used outside of the WLL platform to gain real-world value of some kind.
In addition to the core data structure, Vouchers add a code
property.
This will be null initially until you redeem it, after which any further requests to retrieve
the benefit will include the full code object (check out the “Redeemed Voucher” request on the right to see this data structure).
Gift Card (Xoxo Product)
Gift Cards provide a mechanism for users to get real world value out of the loyalty programme without any fulfilment considerations for yourself as a business.
As soon as a user purchases a gift card reward the Benefit contains the details of how to make use of it.
Since gift cards are a closed-loop system you are not able to know when a user has fully made use of the whole value initially added to the gift card.
For this reason WLL recommends you present UI for the user to call the redeem method for any gift card benefit
at a time of their choosing, this will set the redeemedAt
timestamp on the benefit and allow you to consider the benefit “closed” and no longer present it to the user.
In addition to the core data structure, Xoxo Products add the following properties:
-
url
: most gift cards will return a redemption URL which provides a ready-made UI explaining to the user how to make use of their gift card. -
pin
: some gift cards will return the unique PIN of the card directly, this will need to be presented to the user within your own UI. -
activationCode
: some gift cards require this separate secret code to activate and make use of it.
For an overview of the various reward and benefit flows please see this section of documentation.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization
Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Benefit to retrieve.
Commands ¶
Reward Purchases ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
X-Impersonate: $user_id
Authorization: Bearer $access_token
Body
{
"rewardId": "9cb2cc08-e033-4200-86a7-95a03ba09462"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"rewardId": {
"type": "string"
}
},
"required": [
"rewardId"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"benefit": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": null,
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"benefit": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"type": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"redeemedAt": {
"type": [
"string",
"null"
]
},
"expiresAt": {
"type": [
"string",
"null"
]
},
"reward": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER",
"GENERIC_OFFER",
"AWIN_PROGRAMME",
"FIDEL_OFFER",
"OLIVE_OFFER",
"WEGIFT_PRODUCT",
"XOXO_PRODUCT"
]
},
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"purchasable": {
"type": "boolean"
},
"summary": {
"type": "string"
},
"defaultLocale": {
"type": "string",
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
}
},
"required": [
"type",
"id",
"name",
"pictureUrl",
"purchasable",
"defaultLocale"
]
},
"owner": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"authIdentifier": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"isRestricted": {
"type": "boolean"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
}
},
"required": [
"id",
"accountNumber",
"authIdentifier",
"isRestricted",
"createdAt",
"updatedAt"
]
},
"burnedAt": {
"type": [
"string",
"null"
]
}
},
"required": [
"id",
"type",
"reward"
]
}
}
}
},
"required": [
"status",
"data"
]
}
Purchase a RewardPOST/commands/purchase_reward
Use this endpoint to purchase a Reward defined within White Label Loyalty.
The concept of “purchasing” is used to represent the flow where a user chooses a specific reward. If the chosen reward defines a points “cost” then the user will only be able to complete the purchase if they have sufficient points and that point value will be deducted from their wallet.
See the Understanding Rewards and Benefits for more information.
This endpoint can either be invoked with a privileged administrative access token or with a user’s own access token.
If using an admin token, you must specify the X-Impersonate
header with the ID of the user you wish to execute the purchase as.
If using a user token, the purchase will naturally be considered executed by the user linked to the enclosed token.
If you wish to purchase a reward on behalf of a team you can use the beneficiaryId
property to provide the ID of the team that should receive the benefit of this purchase.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with update:users
scope
Non-compliant requests will be rejected.
Benefit Redemptions ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"benefitId": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"position": {
"longitude": 170.9021,
"latitude": 80.9021
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"benefitId": {
"type": "string"
},
"position": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
}
},
"required": [
"benefitId"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"benefit": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"type": "VOUCHER",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": "2018-06-10T21:07:01.219Z",
"expiresAt": "2019-06-08T08:57:47.198Z",
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
},
"owner": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "any string",
"isRestricted": false,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
}
},
"burnedAt": "2021-06-08T08:57:47.198Z"
},
"code": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": "2018-06-08T08:57:47.198Z",
"validatedAt": "2018-06-08T08:57:47.198Z",
"value": "123ABC"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"benefit": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"type": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"redeemedAt": {
"type": [
"string",
"null"
]
},
"expiresAt": {
"type": [
"string",
"null"
]
},
"reward": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER",
"GENERIC_OFFER",
"AWIN_PROGRAMME",
"FIDEL_OFFER",
"OLIVE_OFFER",
"WEGIFT_PRODUCT",
"XOXO_PRODUCT"
]
},
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"purchasable": {
"type": "boolean"
},
"summary": {
"type": "string"
},
"defaultLocale": {
"type": "string",
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
}
},
"required": [
"type",
"id",
"name",
"pictureUrl",
"purchasable",
"defaultLocale"
]
},
"owner": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"authIdentifier": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"isRestricted": {
"type": "boolean"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
}
},
"required": [
"id",
"accountNumber",
"authIdentifier",
"isRestricted",
"createdAt",
"updatedAt"
]
},
"burnedAt": {
"type": [
"string",
"null"
]
}
},
"required": [
"id",
"type",
"reward"
]
},
"code": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"redeemedAt": {
"type": [
"string",
"null"
]
},
"validatedAt": {
"type": [
"string",
"null"
]
},
"value": {
"type": "string"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"value"
]
}
}
}
},
"required": [
"status",
"data"
]
}
Redeem a BenefitPOST/commands/redeem_benefit
Use this endpoint to redeem a benefit. See the Understanding rewards and benefits for more information.
The position
property is an optional property. You can pass the beneficiary user’s current position geo-coordinates to enable integration with the venues module.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with update:users
scope
Non-compliant requests will be rejected.
Voucher Validations ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"code": "625735",
"userIdentifier": "1003607",
"venueIdentifier": "71231",
"position": {
"longitude": 170.9021,
"latitude": 80.9021
},
"allowFutureValidation": true
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"code": {
"type": "string"
},
"userIdentifier": {
"type": "string"
},
"venueIdentifier": {
"type": "string"
},
"position": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
},
"allowFutureValidation": {
"type": "boolean"
}
},
"required": [
"code"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"isValid": true,
"voucher": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"redeemedAt": "2018-06-08T08:57:47.198Z",
"validatedAt": "2018-06-08T08:57:47.198Z"
},
"reward": {
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"price": 0,
"priority": 0,
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"tier": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Category Name",
"description": "This is an example description",
"priority": 1,
"parent": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"pictureUrl": ""
},
"discounts": [
{
"type": "FIXED",
"value": 1000,
"sku": "321ZA",
"productSku": "AZ123"
}
]
},
"discounts": [
{
"type": "FIXED",
"value": 1000,
"sku": "321ZA",
"productSku": "AZ123"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"isValid": {
"type": "boolean"
},
"voucher": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"redeemedAt": {
"type": "string"
},
"validatedAt": {
"type": "string"
}
},
"required": [
"id",
"createdAt",
"redeemedAt",
"validatedAt"
]
},
"reward": {
"type": "object",
"properties": {
"type": {
"type": "string",
"enum": [
"VOUCHER",
"GENERIC_OFFER",
"AWIN_PROGRAMME",
"FIDEL_OFFER",
"OLIVE_OFFER",
"WEGIFT_PRODUCT",
"XOXO_PRODUCT"
]
},
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"purchasable": {
"type": "boolean"
},
"summary": {
"type": "string"
},
"defaultLocale": {
"type": "string",
"enum": [
"en",
"fr",
"de",
"it",
"es",
"nl",
"ar",
"tr"
]
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"price": {
"type": "number"
},
"priority": {
"type": "number"
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"tier": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
}
},
"required": [
"id",
"name",
"priority"
]
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
},
"pictureUrl": {
"type": "string"
}
}
},
"discounts": {
"type": "array"
}
},
"required": [
"type",
"id",
"name",
"pictureUrl",
"purchasable",
"defaultLocale",
"createdAt",
"updatedAt",
"price",
"priority",
"availability",
"discounts"
]
},
"discounts": {
"type": "array"
}
},
"required": [
"isValid",
"voucher",
"reward"
]
}
},
"required": [
"status",
"data"
]
}
Validate a Voucher CodePOST/commands/validate_voucher
Use this endpoint to check whether a voucher redemption code is valid for use by the specified user.
The code
field is mandatory, the userIdentifier
field is optional but recommended where possible
since it allows validating that the code also belongs to the specified user.
The user identifier should be either the user.authIdentifier
or the user.accountNumber
.
The venueIdentifier
and position
fields are optional, however at least one should be provided to support validating venue-locked rewards. If neither is provided any validation requests for vouchers of venue-locked rewards will be rejected since the system will not be able to verify if the redemption is occuring at a valid venue.
The venueIdentifier
should be either a valid venue.externalIdentifier
or venue.id
.
The position
should be the geocoordinates of the venue where the redemption is occurring.
The allowFutureValidation
allows you to validate a code that exists in our system and hasn’t already been validated (e.g. applying a discount at a PoS). Use allowFutureValidation: true
in the body to not burn the voucher until you call it again without that option (e.g. checking if the voucher is valid and showing the discount, but not burning it until the user checks out). If this field is not specified, then it will default to true.
Applying Discounts
The discount objects returned in this response represent how the redeemed voucher should be applied to the basket. Only the discounts relevant to the indicated venue will be returned.
Discount objects may contain sku
and productSku
fields.
These fields are intended to represent the SKU of the discount itself (if any) and the SKU of the product on which the discount should be applied (if any).
Absolute Discounts
A discount with type: 'ABSOLUTE'
indicates that the product or basket price should be adjusted relative to the price before discounting.
The value
is an integer which represents the adjustment amount in the lowest denomination of the currency.
For example given a basket price of £1.50, applying an absolute discount of value: 100
would result in a new basket price of £0.50.
Percentage Discounts
A discount with type: 'PERCENTAGE'
indicates that the product or basket price should be adjusted by the given percentage of the price before discounting.
The value
is an integer which represents the percentage 0-100. For example given a basket price of £1.50, applying a percentage discount of value: 10
would result in a new basket price of £1.35.
Fixed Discounts
A discount with type: 'FIXED'
indicates that the product or basket price should be set to the given the price.
The value
is an integer which represents new price to set, in the lowest denomination of the currency.
For example given a basket price of £1.50, applying an fixed discount of value: 100
would result in a new basket price of £1.00.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with validate:vouchers
scope. Non-compliant requests will be rejected.
Badge Awards ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"badgeId": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"beneficiaryId": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"comment": "Great work!"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"badgeId": {
"type": "string"
},
"beneficiaryId": {
"type": "string"
},
"comment": {
"type": "string"
}
},
"required": [
"badgeId",
"beneficiaryId"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"badge": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
},
"comment": "Great work!"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"badge": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"status": {
"type": "string",
"enum": [
"ACTIVE",
"INACTIVE",
"ARCHIVED"
]
},
"details": {
"type": "array"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"internalName",
"priority",
"details"
]
},
"comment": {
"type": "string"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"badge"
]
}
},
"required": [
"status",
"data"
]
}
Award a badgePOST/commands/award_badge
Use this endpoint to award a Badge to a specific user.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with at least update:users
OR update:teams
scope depending on whether you’re targeting a User
or a Team
. Non-compliant requests will be rejected.
Referral Campaigns ¶
Specific User Referral Code ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {}
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Generate Referral Code for Specific UserPOST/users/{id}/referral_code
Use this endpoint to generate a referral code for a specific user. This should ideally be called when the user is ready to share their referral code with their friends.
Referral code data returned in the response has the following keys of interest:
inviteCode
: this represents a referral code (eg: REF200) that a new user can manually copy/paste in a referral code field during sign up.inviteLink
: this represents a referral link (eg: custom.app.link/X22TUV) that can simply be clicked to first redirect the new user to an app store and then to automatically pre-fill the referral code field during sign up.
In most cases, the inviteCode
and the inviteLink
generated would be the same link. The user can copy and paste this link during sign up if needed, and use it like a manually usable referral code.
To keep it simple, in such cases where both the values are the same, you can just use the inviteLink
for sharing purposes.
The campaign
property contains metadata about the referral campaign that was previously setup using WLL’s console.
The campaign
property itself consists of properties like the name
of the campaign for admin purposes, and linkConfig
.
The data contained in this linkConfig
property can be used to construct a message that can be shared by the user along with the referral code. For eg, the title
property can be used as the title of the message, and description
as the body of the message.
The referrer
property simply contains the details of the User this referral code is being generated for.
Once the referral code/link is generated for the user, subsequent requests to this endpoint can either return the existing referral code or generate a new one depending on some factors. One of the factors is whether a new referral campaign was active at the time of generation of the referral code or not.
The referral code/link generated is unique for the referrer user, and a single referrer user can have many referral codes/links generated for them over their lifetime.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with at least admin:users
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the User for whom the referral code should be generated.
Active User Referral Code ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {}
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Generate Referral Code for Active UserPOST/users/me/referral_code
Generate a referral code for the currently authenticated user.
See the Specific User Referral Code documentation for more information.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
Handle User's Referral Code Activity ¶
This can be either a Registration or a Click activity
For more information regarding handling Registration, see Register a new User
List Referral Codes ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z",
"referrals": [
{
"sourceUserId": "9cb2cc08-e033-4200-86a7-95a03ba04875",
"source": "EMAIL",
"userAgent": "IOS",
"referralCode": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
},
"registeredUser": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"referralTrancheStatuses": [
{
"trancheId": "9cb2cc08-e033-4200-86a7-95a03ba06845",
"activations": 0,
"isCompleted": false
}
]
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array",
"items": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"referrals": {
"type": "array"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
}
},
"required": [
"status"
]
}
List all Referral Codes for a campaignGET/campaigns/{campaign_id}/referral_codes
Get a list of all referral codes for a specific campaign.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- campaign_id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the campaign to query.
Referral Codes ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z",
"referrals": [
{
"sourceUserId": "9cb2cc08-e033-4200-86a7-95a03ba04875",
"source": "EMAIL",
"userAgent": "IOS",
"referralCode": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
},
"registeredUser": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"referralTrancheStatuses": [
{
"trancheId": "9cb2cc08-e033-4200-86a7-95a03ba06845",
"activations": 0,
"isCompleted": false
}
]
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"referrals": {
"type": "array"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a Referral CodeGET/campaigns/{campaign_id}/referral_codes/{referral_code}
Retrieve a referral code
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- campaign_id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the campaign the referral code belongs to.
- referral_code
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09786ID of the referral code to retrieve.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z",
"referrals": [
{
"sourceUserId": "9cb2cc08-e033-4200-86a7-95a03ba04875",
"source": "EMAIL",
"userAgent": "IOS",
"referralCode": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
},
"registeredUser": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"referralTrancheStatuses": [
{
"trancheId": "9cb2cc08-e033-4200-86a7-95a03ba06845",
"activations": 0,
"isCompleted": false
}
]
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"referrals": {
"type": "array"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Update a Referral CodePATCH/campaigns/{campaign_id}/referral_codes/{referral_code}
Update a referral code
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- campaign_id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the campaign the referral code belongs to.
- referral_code
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09786ID of the referral code to update.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z",
"referrals": [
{
"sourceUserId": "9cb2cc08-e033-4200-86a7-95a03ba04875",
"source": "EMAIL",
"userAgent": "IOS",
"referralCode": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
},
"registeredUser": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"referralTrancheStatuses": [
{
"trancheId": "9cb2cc08-e033-4200-86a7-95a03ba06845",
"activations": 0,
"isCompleted": false
}
]
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"referrals": {
"type": "array"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Delete a Referral CodeDELETE/campaigns/{campaign_id}/referral_codes/{referral_code}
Delete a referral code
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- campaign_id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the campaign the referral code belongs to.
- referral_code
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09786ID of the referral code to update.
Create a Referral ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"clickCount": 1,
"source": "EMAIL",
"sourceUserId": "Hello, world!",
"userAgent": "IOS",
"ipAddress": "Hello, world!"
}
Schema
{
"type": "object",
"properties": {
"clickCount": {
"type": "number"
},
"source": {
"enum": [
"EMAIL",
"FACEBOOK",
"TWITTER",
"LINKEDIN",
"OTHER"
]
},
"sourceUserId": {
"type": "string"
},
"userAgent": {
"enum": [
"IOS",
"ANDROID",
"WEB",
"UNKNOWN"
]
},
"ipAddress": {
"type": "string"
}
},
"required": [
"clickCount",
"source",
"sourceUserId"
],
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z",
"referrals": [
{
"sourceUserId": "9cb2cc08-e033-4200-86a7-95a03ba04875",
"source": "EMAIL",
"userAgent": "IOS",
"referralCode": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {}
},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
},
"registeredUser": {
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
},
"referralTrancheStatuses": [
{
"trancheId": "9cb2cc08-e033-4200-86a7-95a03ba06845",
"activations": 0,
"isCompleted": false
}
]
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {
"isRestricted": {
"type": "boolean"
},
"profile": {
"type": "object",
"properties": {
"familyName": {
"type": "string"
},
"givenName": {
"type": "string"
},
"emailAddress": {
"type": "string"
},
"telephoneNumber": {
"type": "string"
},
"gender": {
"type": "string",
"enum": [
"UNKNOWN",
"MALE",
"FEMALE",
"OTHER"
]
},
"birthdate": {
"type": "string"
},
"streetAddress": {
"type": "string"
},
"city": {
"type": "string"
},
"postcode": {
"type": "string"
},
"region": {
"type": "string"
},
"country": {
"type": "string"
},
"pictureUrl": {
"type": "string"
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
}
},
"required": [
"familyName",
"givenName",
"emailAddress"
]
},
"externalIdentifier": {
"type": "string"
},
"authIdentifier": {
"type": "string"
},
"linkedVenues": {
"type": "array"
},
"id": {
"type": "string"
},
"accountNumber": {
"type": "number"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"flags": {
"type": "object",
"properties": {
"genderProvided": {
"type": "boolean"
}
}
},
"referralCodeUsedForRegistration": {
"type": "object",
"properties": {
"inviteCode": {
"type": "string"
},
"inviteLink": {
"type": "string"
},
"campaign": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"linkConfig": {
"type": "object",
"properties": {
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"desktopUrl": {
"type": "string"
},
"imageUrl": {
"type": [
"string",
"null"
]
},
"iosUrl": {
"type": [
"string",
"null"
]
},
"androidUrl": {
"type": [
"string",
"null"
]
}
},
"required": [
"title",
"description",
"desktopUrl"
]
},
"isActive": {
"type": "boolean"
},
"tranches": {
"type": "array"
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"linkConfig",
"isActive",
"id"
]
},
"referrer": {
"type": [
"object",
"null"
],
"properties": {}
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"isRestricted",
"profile",
"authIdentifier",
"id",
"accountNumber",
"createdAt",
"updatedAt"
]
},
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"referrals": {
"type": "array"
}
},
"required": [
"inviteCode",
"inviteLink",
"campaign",
"id"
]
}
},
"required": [
"status",
"data"
]
}
Create a ReferralPOST/campaigns/{campaign_id}/referral_codes/{referral_code}/referral
Creates a referral for a specific referral code
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:users
scope. Non-compliant requests will be rejected.
- campaign_id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the campaign the referral code belongs to.
- referral_code
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09786ID of the referral code to update.
Venues ¶
Venues Collection ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all VenuesGET/venues{?limit,skip,sort,order,filter}
Get a list of all venues defined within your tenant.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: nameThe property to use for sorting.
Choices:
name
address
externalId
createdAt
updatedAt
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"externalIdentifier": "anyidentifier123",
"attributes": {
"key": "value"
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"name": {
"type": "string"
},
"address": {
"type": "string"
},
"postcode": {
"type": "string"
},
"coordinates": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name"
]
}
},
"required": [
"status",
"data"
]
}
Create a VenuePOST/venues
Create a new Venue.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:venues
scope. Non-compliant requests will be rejected.
Individual Venue ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"name": {
"type": "string"
},
"address": {
"type": "string"
},
"postcode": {
"type": "string"
},
"coordinates": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a VenueGET/venues/{id}
Retrieve the details of a single Venue.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue to Retrieve.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"shouldCalculateCoordinates": false
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"name": {
"type": "string"
},
"address": {
"type": "string"
},
"postcode": {
"type": "string"
},
"coordinates": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name"
]
}
},
"required": [
"status",
"data"
]
}
Update a VenuePATCH/venues/{id}
Update the details of a single Venue.
If you are updating the address of the venue and you want the system to automatically calculate
new coordinates based on the address you can pass shouldCalculateCoordinates: true
. This behaviour is opt-in.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:venues
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue to update.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"externalIdentifier": "anyidentifier123",
"name": "starbucks",
"address": "12 Road Street, Leeds, UK",
"postcode": "LS1 5PZ",
"coordinates": {
"longitude": 170.9021,
"latitude": 80.9021
},
"availability": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"category": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
},
"attributes": {
"key": "value"
},
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"externalIdentifier": {
"type": "string"
},
"name": {
"type": "string"
},
"address": {
"type": "string"
},
"postcode": {
"type": "string"
},
"coordinates": {
"type": "object",
"properties": {
"longitude": {
"type": "number"
},
"latitude": {
"type": "number"
}
},
"required": [
"longitude",
"latitude"
]
},
"availability": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
},
"attributes": {
"type": "object",
"properties": {
"key": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name"
]
}
},
"required": [
"status",
"data"
]
}
Delete a VenueDELETE/venues/{id}
Delete a Venue. Note that the venue will be marked as no longer available, rather than being fully removed from the platform and will remain accessible via the Loyalty Console.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:venues
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue to delete.
Venue Rewards ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"type": "VOUCHER",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"name": "Free Cup of Coffee",
"pictureUrl": "https://example.com/pic.jpg",
"purchasable": false,
"summary": "Example summary",
"defaultLocale": "en"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List Venue RewardsGET/venues/{id}/rewards{?limit,skip,sort,order,filter}
Get a list of any rewards which have been explicitly assigned to this venue.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue for which rewards should be retrieved.
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: nameThe property to use for sorting.
Choices:
priority
price
value
name
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Venue Users ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"isRestricted": false,
"profile": {
"familyName": "Doe",
"givenName": "Jane",
"emailAddress": "jane.doe@example.com",
"telephoneNumber": "01437 522633",
"gender": "UNKNOWN",
"birthdate": "1970-01-01",
"streetAddress": "12 Bedford Street",
"city": "Leeds",
"postcode": "LS1 5PZ",
"region": "West Yorkshire",
"country": "GB",
"pictureUrl": "https://example.com/pic.jpg",
"attributes": {
"key": "value"
}
},
"externalIdentifier": "any string",
"authIdentifier": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"linkedVenues": [
{
"id": "8d68cb91-202a-4c62-a867-8f9a8a1e2f50"
}
],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"accountNumber": 1000001,
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"flags": {
"genderProvided": false
},
"referralCodeUsedForRegistration": {
"inviteCode": "custom.app.link/XYI123sSD",
"inviteLink": "custom.app.link/XYI123sSD",
"campaign": {
"name": "Holidays Referral Campaign",
"linkConfig": {
"title": "Join us!",
"description": "Your friend has invited you to checkout this app",
"desktopUrl": "www.custom-domain.com",
"imageUrl": "imgur.com/12323",
"iosUrl": "iosappstorelink",
"androidUrl": "androidplaystorelink"
},
"isActive": true,
"tranches": [],
"id": "9cb2cc08-e033-4200-86a7-95a03ba09400",
"createdAt": "2021-05-08T08:57:47.198Z",
"updatedAt": "2021-05-08T08:57:47.198Z"
},
"referrer": {},
"id": "9cb2cc08-e033-4200-86a7-95a03ba09461",
"createdAt": "2021-06-08T08:57:47.198Z",
"updatedAt": "2021-06-08T08:57:47.198Z"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List Venue UsersGET/venues/{id}/users{?limit,skip,sort,order,filter}
Get a list of any users which have been explicitly linked to this venue.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:tiers
scope. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue for which rewards should be retrieved.
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: idThe property to use for sorting.
Choices:
id
givenName
familyName
emailAddress
externalId
createdAt
updatedAt
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Venue Categories ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all Venue CategoriesGET/venue_categories{?limit,skip,sort,order,filter}
Get a list of all defined venue categories.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: priorityThe property to use for sorting.
Choices:
id
createdAt
updatedAt
name
priority
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: priority:(gt:10)O-Rison encoded filter string
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
}
Schema
{
"type": "object",
"properties": {
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
}
},
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
}
},
"required": [
"status",
"data"
]
}
Creating Venue CategoriesPOST/venue_categories
API to create a Venue Category
Specific Venue Category ¶
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"name": "Restaurants",
"description": "This is an example description",
"priority": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"priority": {
"type": "number"
},
"parent": {
"type": "string"
}
}
}
},
"required": [
"status",
"data"
]
}
Update a Venue CategoryPATCH/venue_categories/{id}
Update the details of the venue category specified by their ID.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with update:venues
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue Category to update.
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"affected": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"affected": {
"type": "number",
"description": "Number of records affected by the delete operation."
}
}
}
},
"required": [
"status",
"data"
]
}
Delete a Venue CategoryDELETE/venue_categories/{id}
The venue category will be deleted. Once deleted the venue category is not accessible. (It is not archived)
Note. Before the category can be deleted all associated venues must be unlinked first.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with delete:venues
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Venue Category to delete.
Tiers ¶
Tiers Collection ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0,
"pointsRequirement": 1000,
"calculation": "CALENDAR_PERIOD",
"accumulationPeriod": "P1Y",
"effectivity": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"createdAt": "2019-04-09T10:02:37.515Z",
"updatedAt": "2019-04-09T10:02:37.515Z",
"earnedPoints": 87,
"attained": false
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List all TiersGET/tiers{?locale,skip,limit,sort,order,filter}
Get a list of all tiers defined within your tenant.
If this method is called with standard user authorization the tier response will include the number of points the user has earned towards this tier plus a simple boolean of whether or not the user has attained the tier.
-
attained
- Boolean indicating whether the user has attained this tier. -
earnedPoints
- Number of qualifying points the user has earned towards this tier as of the time of the request. -
pointsRequirement
- Number of qualifying points required to attain this tier.
If the calculation is CALENDAR_PERIOD
then the accumulationPeriod
property indicates the qualification period for this tier. The value is a string in ISO 8601 period format. P1Y
- annual, P1M
- monthly.
Tier status will expire at the end of the subsequent period unless the user meets the criteria in the current period.
You can see the different response data by switching between the two defined requests on the right.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with admin:tiers
scope
Details are explained above. Non-compliant requests will be rejected.
Localization
This resource can have multiple different user-facing details defined for different locales.
By default, this endpoint will return the details provided by the default locale, however you may pass the locale
query parameter to receive the response details defined for that specified locale.
If there are no defined details for the specified locale, the response will use the default locale.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- locale
string
(optional) Example: enWhich locale to load for the Tier’s details. Default locale will be returned if not provided.
Choices:
en
fr
de
it
es
nl
ar
tr
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: nameThe property to use for sorting.
Choices:
name
points
createdAt
updatedAt
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"priority": 0,
"pointsRequirement": 1000,
"calculation": "LIFETIME_EARN",
"accumulationPeriod": "P1Y",
"defaultLocale": "en",
"details": [
{
"locale": "en",
"name": "Gold",
"description": "This is a description",
"artworkUrl": "https://google.com/logo.gif"
}
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0,
"pointsRequirement": 1000,
"calculation": "CALENDAR_PERIOD",
"accumulationPeriod": "P1Y",
"effectivity": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"createdAt": "2019-04-09T10:02:37.515Z",
"updatedAt": "2019-04-09T10:02:37.515Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
},
"pointsRequirement": {
"type": "number"
},
"calculation": {
"type": "string",
"enum": [
"CALENDAR_PERIOD",
"LIFETIME_EARN"
]
},
"accumulationPeriod": {
"type": "string",
"enum": [
"P1Y",
"P1M"
]
},
"effectivity": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name",
"priority",
"pointsRequirement",
"calculation"
]
}
},
"required": [
"status",
"data"
]
}
Create a TierPOST/tiers
Create a new Tier.
The details
array should contain objects specifying the user-facing details for one or more locales.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:tiers
scope. Non-compliant requests will be rejected.
Individual Tier ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0,
"pointsRequirement": 1000,
"calculation": "CALENDAR_PERIOD",
"accumulationPeriod": "P1Y",
"effectivity": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"createdAt": "2019-04-09T10:02:37.515Z",
"updatedAt": "2019-04-09T10:02:37.515Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
},
"pointsRequirement": {
"type": "number"
},
"calculation": {
"type": "string",
"enum": [
"CALENDAR_PERIOD",
"LIFETIME_EARN"
]
},
"accumulationPeriod": {
"type": "string",
"enum": [
"P1Y",
"P1M"
]
},
"effectivity": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name",
"priority",
"pointsRequirement",
"calculation"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a TierGET/tiers/{id}{?locale}
Retrieve the details of a single Tier.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:tiers
scope. Non-compliant requests will be rejected.
Localization
This resource can have multiple different user-facing details defined for different locales.
By default, this endpoint will return the details provided by the default locale, however you may pass the locale
query parameter to receive the response details defined for that specified locale.
If there are no defined details for the specified locale, the response will use the default locale.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Tier to Retrieve.
- locale
string
(optional) Example: enWhich locale to load for the Tier’s details. Default locale will be returned if not provided.
Choices:
en
fr
de
it
es
nl
ar
tr
Headers
Content-Type: application/json
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"pointsRequirement": 1000,
"calculation": "LIFETIME_EARN",
"accumulationPeriod": "P1Y",
"defaultLocale": "en",
"details": [
{
"locale": "en",
"name": "Gold",
"description": "This is a description",
"artworkUrl": "https://google.com/logo.gif"
}
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0,
"pointsRequirement": 1000,
"calculation": "CALENDAR_PERIOD",
"accumulationPeriod": "P1Y",
"effectivity": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"createdAt": "2019-04-09T10:02:37.515Z",
"updatedAt": "2019-04-09T10:02:37.515Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
},
"pointsRequirement": {
"type": "number"
},
"calculation": {
"type": "string",
"enum": [
"CALENDAR_PERIOD",
"LIFETIME_EARN"
]
},
"accumulationPeriod": {
"type": "string",
"enum": [
"P1Y",
"P1M"
]
},
"effectivity": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name",
"priority",
"pointsRequirement",
"calculation"
]
}
},
"required": [
"status",
"data"
]
}
Update a TierPATCH/tiers/{id}
Update the details of a single Tier.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:tiers
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Tier to update.
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "7fbfb45b-4ee0-471a-a464-67ca580eed7c",
"name": "Gold",
"description": "This is an example",
"artworkUrl": "https://google.com/logo.gif",
"priority": 0,
"pointsRequirement": 1000,
"calculation": "CALENDAR_PERIOD",
"accumulationPeriod": "P1Y",
"effectivity": {
"start": "2018-06-08T18:19:10.878Z",
"end": "2018-06-09T18:19:10.878Z"
},
"createdAt": "2019-04-09T10:02:37.515Z",
"updatedAt": "2019-04-09T10:02:37.515Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
},
"priority": {
"type": "number"
},
"pointsRequirement": {
"type": "number"
},
"calculation": {
"type": "string",
"enum": [
"CALENDAR_PERIOD",
"LIFETIME_EARN"
]
},
"accumulationPeriod": {
"type": "string",
"enum": [
"P1Y",
"P1M"
]
},
"effectivity": {
"type": "object",
"properties": {
"start": {
"type": "string"
},
"end": {
"type": "string"
}
}
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"id",
"name",
"priority",
"pointsRequirement",
"calculation"
]
}
},
"required": [
"status",
"data"
]
}
Delete a TierDELETE/tiers/{id}
Delete a Tier. Note that the tier will be marked as no longer available, rather than being fully removed from the platform and will remain accessible via the Loyalty Console.
🔒 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with admin:tiers
scope. Non-compliant requests will be rejected.
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Tier to delete.
Payouts ¶
Payouts APIs are used to enable user to convert their points into real money.
Currently we support payouts via Stripe Payout(beta)
Before using the APIs, your tenant must add the relevant extension in the integrations section of the console.
User Registration ¶
Headers
Content-Type: application/json
Body
{
"returnUrl": "https:xxxxx",
"refreshUrl": "https:xxxxx",
"Headers": "Hello, world!"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"returnUrl": {
"type": "string",
"description": "The URL to which the user is redirected after the Stripe payout registration is completed"
},
"refreshUrl": {
"type": "string",
"description": "The URL to which the user is redirected is the Stripe registration url is not valid"
},
"Headers": {
"type": "string",
"description": "Authorization: Bearer $access_token\nX-Impersonate: $wll_user_id\nX-Api-Key: $api_key"
}
}
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"url": "https:xxxx"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"url": {
"type": "string",
"description": "Stripe URL, which is to be used by the user to register their bank account"
}
},
"required": [
"url"
]
}
},
"required": [
"status",
"data"
]
}
User RegistrationPOST/payouts/register
Stripe Payout
Use this endpoint to enable a user to register their bank account on Stripe.
This API requires two parameters: returnUrl
and refreshUrl
.
The returnUrl
is the URL to which the user is redirected after completing the registration, while the refreshUrl
is the URL to which the user is redirected if the Stripe URL becomes invalid.
In return, the API provides a Stripe URL (data.url
) for the user to complete their bank account registration.
General Information
This endpoint can either be invoked with a privileged administrative access token or with a user’s own access token.
If using an admin token, you must specify the X-Impersonate
header with the ID of the user you wish to register the account for.
If using a user token, the bank account registration will be initiated for the user linked to the enclosed token.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with create:payouts
scope
Details are explained above.
Non-compliant requests will be rejected.
Registration Info ¶
Headers
Content-Type: application/json
Authorization: Bearer $access_token
X-Impersonate: $wll_user_id
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"points": 0,
"conversionRate": 0,
"currencyCode": "usd",
"minimumPayoutAmount": 0,
"registrationStatus": "INPROGRESS"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"points": {
"type": "number",
"description": "The number of points user has in their wallet"
},
"conversionRate": {
"type": "number",
"description": "This is the conversion rate which is defined while registering for the Stripe Payout extension. To be used for performing the payout."
},
"currencyCode": {
"type": "string",
"enum": [
"usd"
],
"description": "This is the currency code which is defined while registering for the Stripe Payout extension. To be used for performing the payout."
},
"minimumPayoutAmount": {
"type": "number",
"description": "This is the minimum payout amount which is defined while registering for the Stripe Payout extension. To be used for performing the payout."
},
"registrationStatus": {
"type": "string",
"enum": [
"INPROGRESS",
"REGISTERED",
"UNREGISTERED",
"FAILED"
]
}
},
"required": [
"points",
"conversionRate",
"currencyCode",
"minimumPayoutAmount",
"registrationStatus"
]
}
},
"required": [
"status",
"data"
]
}
Registration InfoGET/payouts/registration_info
Use this endpoint to get the status of user registration and other details that would help initiate a payout.
This endpoint can either be invoked with a privileged administrative access token or with a user’s own access token.
If using an admin token, you must specify the X-Impersonate
header with the ID of the user you wish to get the details for.
If using a user token, the registration info would be sent for the user linked to the enclosed token.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with read:payouts
scope
Details are explained above.
Non-compliant requests will be rejected.
Request Payout ¶
Headers
Content-Type: application/json
Body
{
"pointsToTransfer": 1,
"Headers": "Hello, world!"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"pointsToTransfer": {
"type": "number",
"description": "The number of points that need to be transferred to the user's bank account after converting it using the conversion rate, which is defined while registering for the Stripe Payout extension"
},
"Headers": {
"type": "string",
"description": "Authorization: Bearer $access_token\nX-Impersonate: $wll_user_id\nX-Api-Key: $api_key"
}
},
"required": [
"pointsToTransfer"
]
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "",
"cancelable": false,
"status": "processing"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string",
"description": "Stripe payment ID"
},
"cancelable": {
"type": "boolean",
"description": "If true the payment is cancelable until the status changes to `posted`"
},
"status": {
"type": "string",
"enum": [
"processing",
"posted",
"canceled",
"failed",
"returned"
]
}
},
"required": [
"id",
"cancelable",
"status"
]
}
},
"required": [
"status",
"data"
]
}
Request PayoutPOST/payouts/pay
Use this endpoint to enable a user to convert their points into real money.
This endpoint can either be invoked with a privileged administrative access token or with a user’s own access token.
If using an admin token, you must specify the X-Impersonate
header with the ID of the user you wish to request the payout for.
If using a user token, the payout would be requested for the user linked to the enclosed token.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with create:payouts
scope
Details are explained above.
Non-compliant requests will be rejected.
Badges ¶
Badges Collection ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List BadgesGET/badges{?locale,skip,limit,sort,order,filter}
Get a list of all badges defined within your tenant.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with admin:tiers
scope
Details are explained above. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- locale
string
(optional) Example: enWhich locale to load for the Badges details. Default locale will be returned if not provided.
Choices:
en
fr
de
it
es
nl
ar
tr
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: createdAtThe property to use for sorting.
Choices:
createdAt
updatedAt
internalName
priority
status
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"internalName": "Example Badge",
"priority": 1,
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50"
}
],
"internalDescription": "An example badge for WLL"
}
Schema
{
"type": "object",
"properties": {
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"details": {
"type": "array",
"items": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"locale": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
}
},
"required": [
"name",
"locale",
"description",
"artworkUrl"
]
}
},
"internalDescription": {
"type": "string"
}
},
"required": [
"internalName",
"priority",
"details"
],
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
],
"internalDescription": "An example badge for WLL"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"status": {
"type": "string",
"enum": [
"ACTIVE",
"INACTIVE",
"ARCHIVED"
]
},
"details": {
"type": "array"
},
"internalDescription": {
"type": "string"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"internalName",
"priority",
"details"
]
}
},
"required": [
"status",
"data"
]
}
Create a BadgePOST/badges
Create a badge within your tenant.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with at least create:badges
scope. Non-compliant requests will be rejected.
Individual Badge ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"status": {
"type": "string",
"enum": [
"ACTIVE",
"INACTIVE",
"ARCHIVED"
]
},
"details": {
"type": "array"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"internalName",
"priority",
"details"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a BadgeGET/badges/{id}{?locale,skip,limit,sort,order,filter}
Get a single badge by its ID.
🔐 Multiple authorization modes
Requests to this method require either:
👤 User Authorization
🔒 Admin Authorization with admin:tiers
scope
Details are explained above. Non-compliant requests will be rejected.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- id
uuid
(required) Example: 9cb2cc08-e033-4200-86a7-95a03ba09462ID of the Badge to retrieve.
- locale
string
(optional) Example: enWhich locale to load for the Badges details. Default locale will be returned if not provided. + Members + en + fr + de + it + es + nl + ar + tr
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: nameThe property to use for sorting. + Members + name + points + createdAt + updatedAt
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Body
{
"internalName": "Example Badge",
"priority": 1,
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50"
}
],
"internalDescription": "An example badge for WLL",
"status": "ACTIVE"
}
Schema
{
"type": "object",
"properties": {
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"details": {
"type": "array",
"items": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"locale": {
"type": "string"
},
"description": {
"type": "string"
},
"artworkUrl": {
"type": "string"
}
},
"required": [
"name",
"locale",
"description",
"artworkUrl"
]
}
},
"internalDescription": {
"type": "string"
},
"status": {
"enum": [
"ACTIVE",
"INACTIVE",
"ARCHIVED"
]
}
},
"required": [
"internalName",
"priority",
"details"
],
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
],
"internalDescription": "An example badge for WLL"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
},
"internalName": {
"type": "string"
},
"priority": {
"type": "number"
},
"status": {
"type": "string",
"enum": [
"ACTIVE",
"INACTIVE",
"ARCHIVED"
]
},
"details": {
"type": "array"
},
"internalDescription": {
"type": "string"
}
},
"required": [
"id",
"createdAt",
"updatedAt",
"internalName",
"priority",
"details"
]
}
},
"required": [
"status",
"data"
]
}
Update a BadgePATCH/badges/
Update a badge within your tenant.
🔐 Admin authorization required
Requests to this method must provide an Authorization
header containing a valid administrative token with at least update:badges
scope. Non-compliant requests will be rejected.
Earned Badges ¶
Headers
X-Api-Key: $api_key
Authorization: Bearer $access_token
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z",
"internalName": "Example Badge",
"priority": 0,
"status": "ACTIVE",
"details": [
{
"name": "Great Work Badge",
"locale": "en",
"description": "A badge for people doing some great work",
"artworkUrl": "https://picsum.photos/50",
"id": "9cb2cc08-e033-4200-86a7-95a03ba09462",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List Earned BadgesGET/badges/earned{?locale,skip,limit,sort,order,filter}
Get a list of all badges earned by users by the authenticated user.
👤 User authorization required
Requests to this method must provide a Authorization
header containing a valid user token signed as per the tenant authentication configuration. Non-compliant requests will be rejected.
- locale
string
(optional) Example: enWhich locale to load for the Badges details. Default locale will be returned if not provided.
Choices:
en
fr
de
it
es
nl
ar
tr
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional)The property to use for sorting.
Choices:
name
createdAt
updatedAt
- order
string
(required) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Settings ¶
Settings Collection ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": [
{
"name": "example.value",
"value": "foobar",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "array"
}
},
"required": [
"status",
"data"
]
}
List SettingsGET/settings{?skip,limit,sort,order,filter}
Get a list of all settings defined within your tenant.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
Filtering
It is possible to apply basic filter conditions to the result set using the filter
query parameter. This parameter accepts an O-Rison encoded JSON object expressing the set of conditions to be applied.
The general format of this filter object (expressed in JSON) is as follows:
{
"field1": { "operator": "value" },
"field2": { "operator": "value" }
}
Where field1
and field2
are field names of this entity, operator
is one of [eq
, neq
, gt
, lt
, in
, has
, like
], and "value"
is the exact value to compare against. The like operator accepts a wild card %
which means it will accept any value in place of it e.g %App%
will find Web App
, the like operator also isn’t case sensitive. The value need not necessarily be a string, the type appropriate for the field should be used. See the O-Rison documentation for help on how to format other types. Dates and datetimes should be passed as ISO 8601 strings.
The same filter encoded as O-Rison would be as follows:
field1:(operator:'value'),field2:(operator:'value')
When using the in
operator the value should be an array.
Arrays are expressed by enclosing a comma separated list of elements within !()
.
For example:
field3:(in:!('value1','value2'))
- limit
number
(optional) Example: 10The number of items to retrieve, defaults to 10 unless otherwise specified. Maximum: 1000.
- skip
number
(optional) Example: 0The number of items to skip before selecting.
- sort
string
(optional) Example: createdAtThe property to use for sorting.
Choices:
createdAt
updatedAt
name
- order
string
(optional) Example: ASCThe order to sort items.
Choices:
ASC
DESC
- filter
string
(optional) Example: createdAt:(gt:'2019-01-01T00:00:00Z')O-Rison encoded filter string
Individual Setting ¶
Headers
X-Api-Key: $api_key
Headers
Content-Type: application/json; charset=utf-8
Body
{
"status": "success",
"data": {
"name": "example.value",
"value": "foobar",
"createdAt": "2018-06-08T08:57:47.198Z",
"updatedAt": "2018-06-08T08:57:47.198Z"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status": {
"type": "string"
},
"data": {
"type": "object",
"properties": {
"name": {
"type": "string"
},
"value": {
"type": "string"
},
"createdAt": {
"type": "string"
},
"updatedAt": {
"type": "string"
}
},
"required": [
"name",
"value",
"createdAt",
"updatedAt"
]
}
},
"required": [
"status",
"data"
]
}
Retrieve a SettingGET/settings/{name}
Get a single setting by its name.
🔑 No authorization required
Requests to this method do not require any authorization other than a valid API key.
- name
string
(required) Example: example.valuename of the setting to retrieve.
Generated by aglio on 18 Jun 2025